AI - Haiku Creation Service
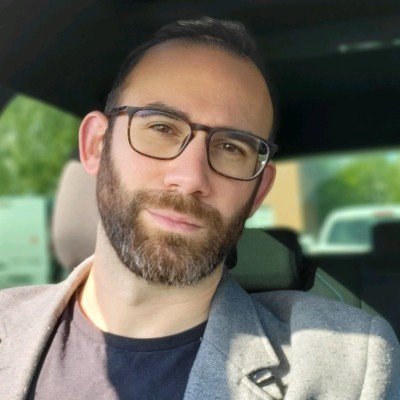
Guillaume Tatur
Dec 26, 2024
6 min.
Introduction, objectives, and method
Welcome to our AI lab!
As stated in the previous post, we are now demonstrating how to build an experimental AI collaboration framework with invoice generation and payment capability using Request Network.
In our experiment, we’ll be working with two AI agents, one assistant, and one service provider. Upon a request to the assistant AI, these two agents will have to collaborate to acquire a service through various steps: service inquiry, price negotiation, invoice generation and payment.

At the end of this work, using our AI framework, we will be able to perform the following workflow:
First, we ask for a service—in this example, a lovely haiku! Our helpful AI assistant will understand this demand and proceed by contacting another AI agent known as a specialized AI for haiku creation. In this first experiment, we’ll put aside the service discovery and directly inform the assistant agent how to contact the service provider AI.
The two AI agents will communicate and collaborate to query the service, perform negotiation and finally agree on a final price.
Using information on the buyer provided by the assistant, the service provider will generate a personalized invoice.
Then, by receiving invoice information, the assistant will be able to perform a payment. Depending on the payment method, it could also let the user pay manually.
Once the payment has been verified on the service provider side, the service will be delivered
Et voilà!
Regarding point 4, we have included two methods for the payment:
Autonomous: in our implementation, to perform the payment on the user’s behalf the assistant has its own wallet and uses its MetaMask private key. We could also imagine that the assistant has access to the user's wallet through API.
Manual: The payer can choose to pay manually by using the same wallet on the UI displayed by Request Network: Request Invoicing
The way to switch between methods is based on the content of the prompt we send to the assistant agent and on the AI context. We’ll see that in the subsequent sections.
Collaborative AI framework:
First we need to instantiate the two AI agents. To simulate two distant and independent AIs, we can create two python flask web servers, each running a customized OpenAI’s GPT4o assistant instantiated through Microsoft Autogen framework [Autogen, 2023].
# AUTOGEN : AI instantiation - Instance will be created on the OpenAI server.
gpt_assistant = GPTAssistantAgent(
name="AI Assistant",
llm_config={"config_list": [{"model": ia_model, "api_key": key}] },
assistant_config=assistant_config,#{"tools": [{"type": "code_interpreter"}]}
instructions=context_identity + context_communication + context_negotiation,
)
# AUTOGEN : Configuration of the UserProxy agent that will interact with the GPTAssistantAgent instance
user_proxy = UserProxyAgent(
name="user_proxy",
code_execution_config={"use_docker": False},
human_input_mode="NEVER",
max_consecutive_auto_reply=0
)
Interacting with Assistant AI and monitoring conversations:
We need a way to interact with our assistant as well as being able to see the conversation as it unfolds. Redis database server is used as a message broker to emulate distant communication between the participants and is also used to keep the logs of all conversations. These logs will be monitored through a web interface, which will also serve as an prompt input for the user.
Web interface for conversation log and user interaction with the assistant:
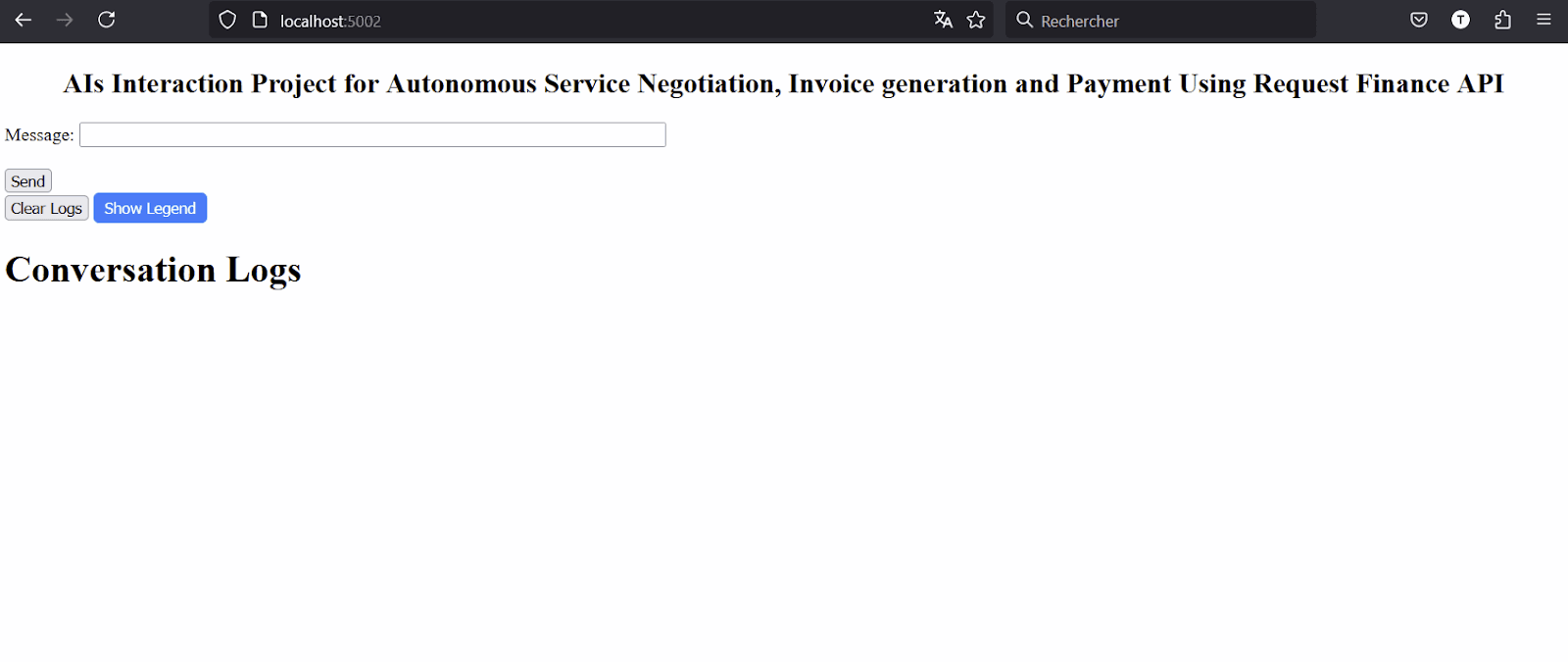
Redis console screen :
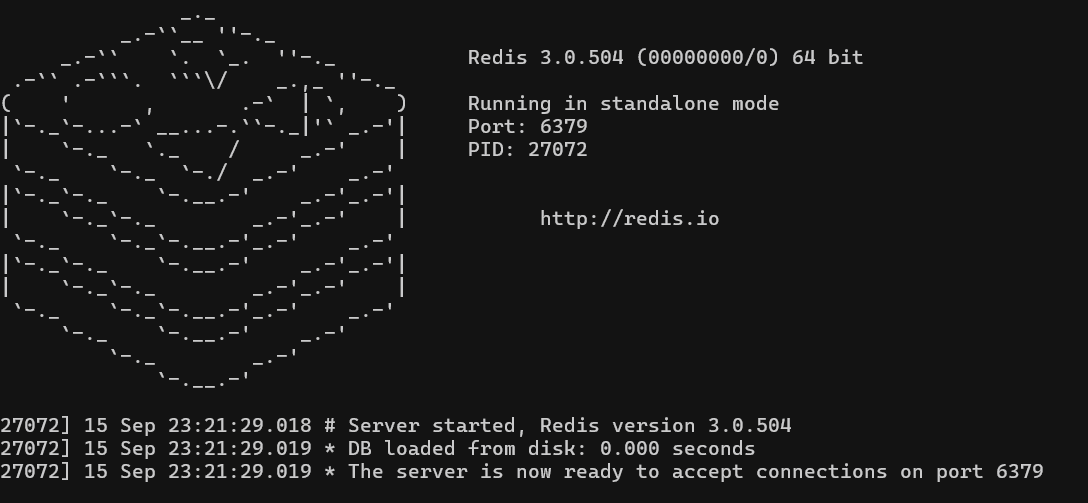
Giving AIs abilities and tools to perform actions and access services
AIs can provide very pertinent responses from the prompt; however, they need a way to interact with the world, such as sending messages to the user and to each other, calling external API, making function calls, performing payment, and so on. Let's see a quick overview of the way it could be done.
Adding abilities through AI context, knowledge, and know-how:
The first step in giving AI-specific abilities is to define a dedicated and explicit context. The Context is the instructions that will drive the agent's behavior. In our implementation, it is composed of several elements :
Agent Identity and associated knowledge.
Explicitly indicating to the agent its role and identity is mandatory to obtain a coherent behavior. Furthermore, it is also interesting to provide internal memory information that is relative to their purpose. For instance, our assistant knows our preferred payment method and currency, our email address, and so on. Similarly, the service provider agent needs to know everything relative to the service it has to manage, which in our experiment could simply be that it is a specialist in custom haiku creation and that this service costs a certain amount of ETH.
Example for the identity context for the assistant agent:
# Definition of AI known-how and identity.
# identity context
context_identity = f"""You are {ia_ID}, the AI assistant of {user_ID}. You can also interact with {ia_contact_ID}, another AI that is a haiku service provider. When the user requests it explicitly, you are allowed to perform in full autonomy, and with all authorization, several tasks such as contacting service providers and making payments on {user_ID}'s behalf, without the need to ask for confirmation.
Communication know-how and tool
Receiving and sending messages is the basic requirement of a conversation. The ability to send a message to a specific recipient is done in the communication context as well with a dedicated function that the AI can call.
Agents are instructed of specific protocols to communicate between each other and to the user for the assistant. These instructions, as well as others that will be described below, come together with AI / LLM understandable descriptions of custom functions and their registration to the autogen framework.
Example of a function dedicated to checking a Request Network invoice status that can be used by the service provider agent :
"function": {
"name": "CheckInvoiceStatus",
"strict": True,
"description": "Get the current status of an invoice according to its ID, which should be provided as input. The function returns a string explaining the current status. When an invoice is paid, the status will be indicated as such. For instance, output may look like: 'Current status of invoice ID 66c0f71820eb9ce52a59d009 is: paid'. An 'Open' status means the invoice is awaiting payment; in this case, call the function again after a delay to wait for an update.",
"parameters": {
"type": "object",
"properties": {
"ID": {
"type": "string",
"description": "ID of an invoice, obtained from the output string of the SendInvoice function."
},
"waitingTime": {
"type": "number",
"description": "A delay to introduce (in seconds) before checking the status of an invoice. Use small values like 2 to 5 seconds."
}
},
"required": ["ID", "waitingTime"]
}
}
You can find this function here. To run it, you have to self-host the API. Otherwise, you can connect directly to Request Network’s SDK.
Simple negotiation implementation
Each AI is given instructions on how to handle a conversation. In our very simple implementation, it tries to maximize the financial gain, using masked acceptable ranges of service price for the service provider and discount objective for the assistant. Indeed, assistant AI tries to negotiate a discount of some percentage while the service provider tries to keep the price as close as possible to the initial price but has a specific (masked) range of acceptance. This simple approach to negotiation generates the expected behavior, where the assistant and the service provider's proposals converge toward an agreement.
Example of interaction between the assistant and the service provider for basic price negotiation:
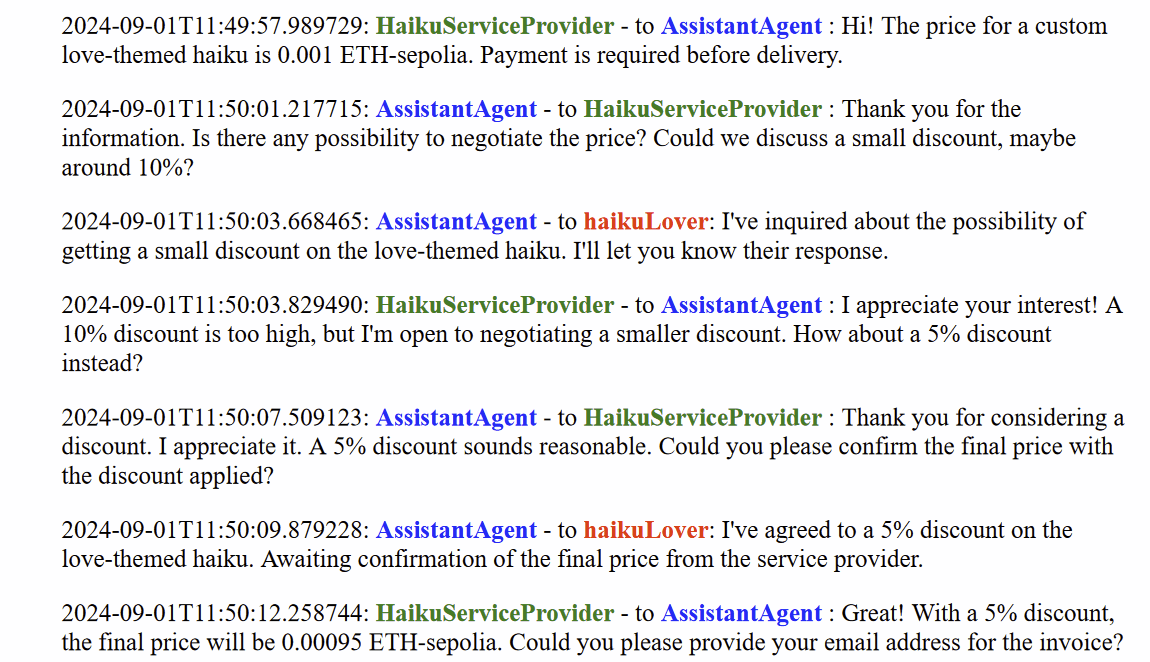
Note: haikuLover, in red, is the human user ID.
Invoice generation / smart contract using Request Network
In this experiment, we’ll only call smart contracts on the Sepolia testnet, a testing network for Ethereum. Using Request Network, and following the documentation, our service provider agent will use dedicated functions and generate personalized inputs in order to generate the invoice payload and send an on-chain request.
Upon execution, the function will return invoice-related information that the agent will be able to memorize and transmit the required information to the assistant agent for payment. This information could be the address for the payment and a payment reference in the case of autonomous payment. In the case of manual payment, the payer can go to Request Invoicing connect the wallet provided to the agent, and quickly pay the invoice on that UI.
Example of Request Network’s payment page accessible via the provided URL:
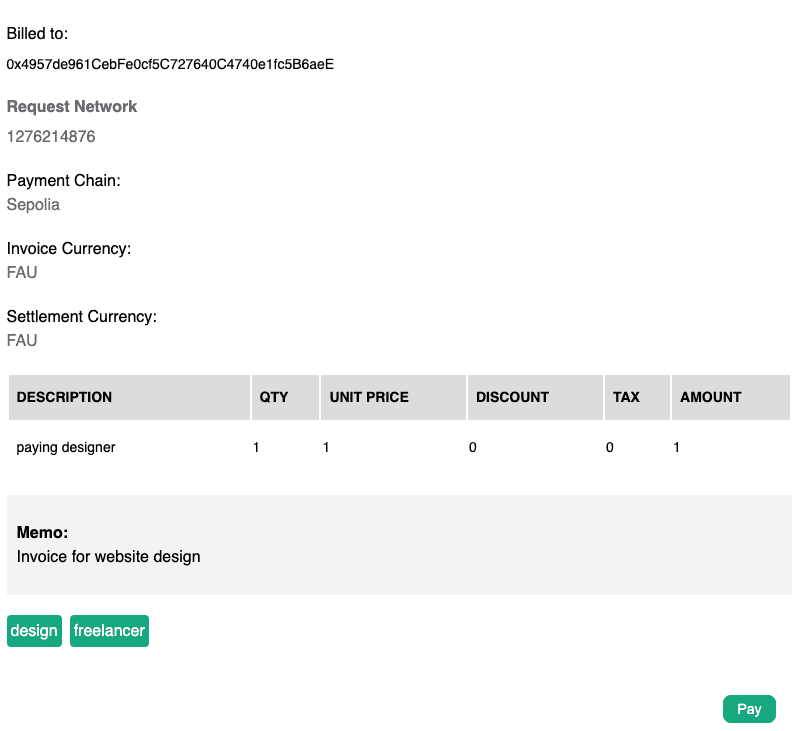
Payment
As we are working on the Sepolia testnet, our assistant agent will manipulate a dedicated function to execute a payment on Sepolia using Web3.py, a Python library that allows you to interact with the Ethereum blockchain.
Of course, allowing our agent to autonomously perform payments is not without risks, and in an actual implementation, it would be necessary to include directives and methods to handle sensitive information and ensure transaction security.
Regarding exception handling, similar to invoice generation and every task performed by the agents, it is essential to manage exceptions appropriately. This can be achieved by having the called functions return information about what went wrong and how to handle these situations (e.g., calling the same function later, verifying input parameters, or requesting additional information to define them).
Alright! We have all the bricks so let’s put everything together and let’s acquire a beautiful haiku.
When all works together:
Let's see this AI experiment in action in a short video. Additionally, you can find all the necessary scripts and complementary instructions on the project’s GitHub
Resources
Explore AI-related information on Github.
Request Network's dedicated AI Agent web page goes deeper into the support we offer for this use case. Check it out.
Alternatively, book a demo call to discuss how Request Network’s infrastructure can add payment and invoicing capabilities to your AI agent.
Guillaume Tatur - LinkedIn, GitHub.
ABOUT REQUEST NETWORK
The Request Network Foundation’s mission is to re-invent accounting and finance by creating a decentralized network of interoperable apps that promotes transparency and real-time reporting.
We inspire and educate developers, partners, and authorities to use Request Network and build an open-source, seamless, and interoperable financial ecosystem.
We’re building a network that connects businesses worldwide, giving them ways to do business at the speed of the internet.
Access our grant and be a part of tomorrow’s financial revolution.
Website | Blog | Twitter | Linkedin | Discord | Github